register_graphql_field
Add a field to a Type in the GraphQL Schema
register_graphql_field( string $type_name, string $field_name, array $config );
Parameters
- $type_name (string): The name of the GraphQL Type in the Schema to register the field to
- $field_name (string): The name of the field. Should be unique to the Type the field is being registered to.
- $config (array): Configuration for the field
- $type (string | array): The name of the GraphQL Type the field will return. The resolve function must return this type.
- For non-null fields:
'type' => [ 'non_null' => 'TypeName' ]
- For listOf fields:
'type' => [ 'list_of' => 'TypeName' ]
- For non-null fields:
- $description (string): Description of the field. This will be used to self-document the schema and should describe to clients how the field should be used.
- $resolve (function): Function that will execute when the field is asked for in a query.
- $type (string | array): The name of the GraphQL Type the field will return. The resolve function must return this type.
Source
File: access-functions.php
Examples
Below are some examples of using the function to extend the GraphQL Schema.
Register a Root Field
This example adds a field to the root of the GraphQL Schema.
add_action( 'graphql_register_types', function() {
register_graphql_field( 'RootQuery', 'testField', [
'type' => 'String',
'description' => __( 'Example field added to the RootQuery Type', 'replace-with-your-textdomain' ),
'resolve' => function( $root, $args, $context, $info ) {
return 'Example string.';
}
] );
});
Example Query
{
testField
}
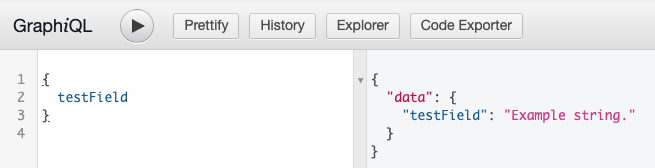
Register a Post Field
This example shows how to register a field to the Post type.
add_action( 'graphql_register_types', function() {
register_graphql_field( 'Post', 'testPostField', [
'type' => 'String',
'description' => __( 'Example field added to the Post Type', 'replace-with-your-textdomain' ),
'resolve' => function( \WPGraphQL\Model\Post $post, $args, $context, $info ) {
return 'Example string with the title of the post: ' . $post->titleRendered;
}
] );
});
Example Query
{
posts(first: 1) {
nodes {
title
testPostField
}
}
}
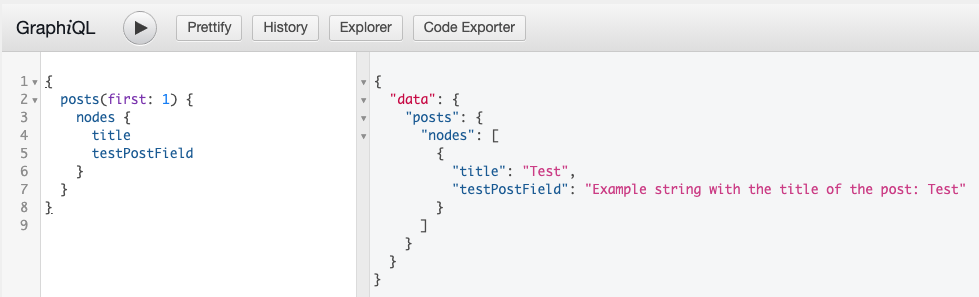
Register a field to an Interface
This example shows how to register a field to an Interface. In this example, a field is registered to the “ContentNode” Interface. This means any Type (any WordPress Post Type) in the Schema that implements the “ContentNode” interface will have the field.
add_action( 'graphql_register_types', function() {
register_graphql_field( 'ContentNode', 'testContentNodeField', [
'type' => 'String',
'description' => __( 'Example field added to the ContentNode Interface', 'replace-with-your-textdomain' ),
'resolve' => function( \WPGraphQL\Model\Post $post_model, $args, $context, $info ) {
return 'Example string with the title of the post: ' . $post->titleRendered;
}
] );
});
Example Query:
{
contentNodes(first: 2, where: {contentTypes: [PAGE, POST]}) {
nodes {
__typename
id
testContentNodeField
}
}
}
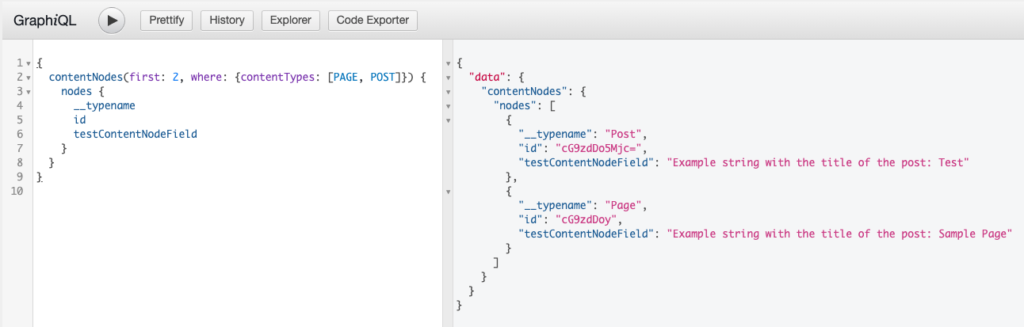